Recently, some users in the telegram group have privately messaged me that he would like to have a design example reference for participating in the IPO subscription with funds strategy. Sometimes a new currency is listed on the exchange and he wants to grab new currencies. This article will design a simple tool strategy for participating in the IPO subscription with funds.
Strategy requirements
For example, a certain exchange, a certain trading pair: XXX_USDT, has not yet listed on the exchange, but it is about to list. A program is needed to keep an eye on this market of XXX_USDT on this exchange. Once the trading pair is listed and ready to trade, it will issue 10 buy orders with a limit, specify the quantity, and hang the orders to grab the currencies. If you can buy, you can complete the task. If you can’t buy, hang on until the orders are filled and the currencies are bought.
The requirement is very simple, but for those who have no programming foundation about the cryptocurrency, they may not be able to start. Next, we will implement it.
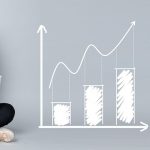
Strategy code
Strategy parameter definition:
Here we define these parameters to control operations, such as placing orders.
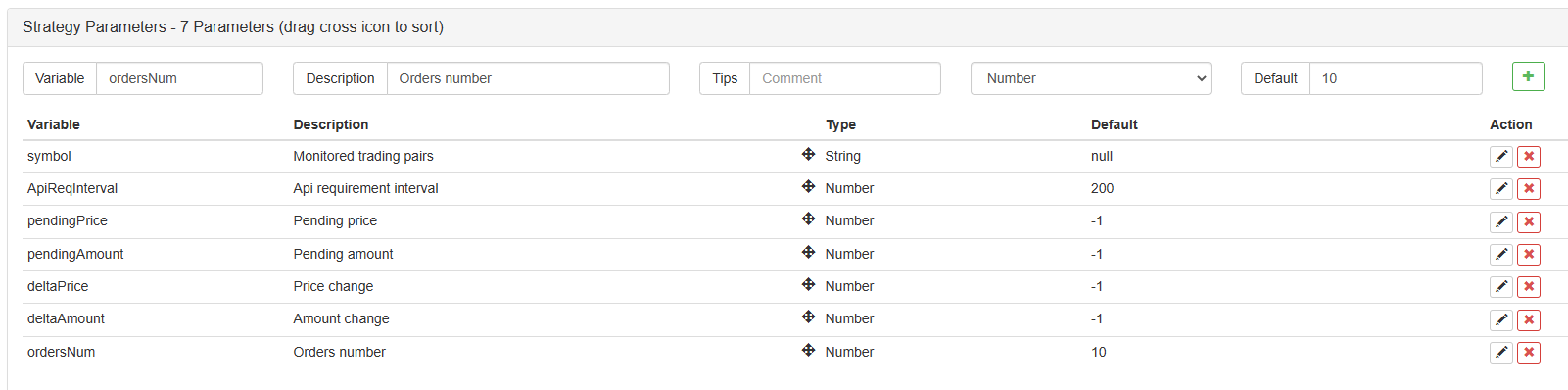
Code implementation:
function pendingOrders(ordersNum, price, amount, deltaPrice, deltaAmount) { var routineOrders = [] var ordersIDs = [] for (var i = 0 ; i < ordersNum ; i++) { var routine = exchange.Go("Buy", price + i * deltaPrice, amount + i * deltaAmount) routineOrders.push(routine) Sleep(ApiReqInterval) } for (var i = 0 ; i < routineOrders.length ; i++) { var orderId = routineOrders[i].wait() if (orderId) { ordersIDs.push(orderId) Log("Successful pending order", orderId) } } return ordersIDs } function main() { if (symbol == "null" || pendingPrice == -1 || pendingAmount == -1 || pendingPrice == -1 || deltaPrice == -1 || deltaAmount == -1) { throw "Parameter setting errors" } exchange.SetCurrency(symbol) // Block error messages SetErrorFilter("GetDepth") while (true) { var msg = "" var depth = exchange.GetDepth() if (!depth || (depth.Bids.length == 0 && depth.Asks.length == 0)) { // No depth msg = "No in-depth data, wait!" Sleep(500) } else { // Obtain the depth Log("Concurrent order!") var ordersIDs = pendingOrders(ordersNum, pendingPrice, pendingAmount, deltaPrice, deltaAmount) while (true) { var orders = _C(exchange.GetOrders) if (orders.length == 0) { Log("The current number of pending orders is 0, stop running") return } var tbl = { type: "table", title: "current pending orders", cols: ["id", "price", "quantity"], rows: [] } _.each(orders, function(order) { tbl.rows.push([order.Id, order.Price, order.Amount]) }) LogStatus(_D(), "\n`" + JSON.stringify(tbl) + "`") Sleep(500) } } LogStatus(_D(), msg) } }
The strategy detects the exchange API and the order book interface. Once the order book data is available, the strategy uses the exchange.Go function to place orders concurrently. After placing an order, the status of the current pending order is cyclically detected. The strategy has not been tested in practice, here is just a code design reference, those who are interested can modify and add functions.
Complete strategy at: https://www.fmz.com/strategy/358383