When testing and debugging the strategy code, or running the robot on the real bot, the exchange interface often reports errors. At this time, go to the exchange interface API document to query the relevant error information. When consulting the exchange API technical customer service, you always need to provide the request message when the error is reported to analyze the cause of the error.
At this time, there is no way to find problems without seeing the message information. In this article, we will discuss two solutions together.
1. Using Python's scapy library to grab packets and print out the request messages sent
First we install the scapy
module
pip3 install scapy
Then we create a python strategy:
from scapy.all import * def Method_print(packet): ret = "\n".join(packet.sprintf("{Raw:%Raw.load%}").split(r"\r\n")) Log(ret) sniff( iface='eth0', prn=Method_print, lfilter=lambda p: "GET" in str(p) or "POST" in str(p), filter="tcp")
Then we create a robot that uses the strategy, and that bot will catch http packets sent from the server of the docker provider it belongs to (that https cannot catch we will have some solutions of this).
Run the packet-catching robot, and then you can use the debugging tool to send requests to let the robot catch packets. In the debugging tool, we write the code that sends the request.
function main(){ // The base address should be set as the address of other http protocols. If the exchange address is not set, it is generally https. In this case, packets cannot be captured exchange.SetBase("http://www.baidu.com") // POST request exchange.IO("api", "POST", "/api/swap/v3/order", "aaa=111&bbb=222") // GET request exchange.SetContractType("swap") exchange.GetTicker() }
Information printed by the package-catching robot:

We can copy out and see the message:
GET request message:
GET /api/swap/v3/instruments/BTC-USD-SWAP/ticker HTTP/1.1 Host: www.baidu.com User-Agent: Mozilla/5.0 (Macintosh; Intel Mac OS X 10_9_3) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/35.0.1916.153 Safari/537.36 Accept-Encoding: gzip
Host: www.baidu.com
is what we changed in order to be able to catch the packet, you can ignore it, the correct one should be Host: www.okex.com
We can see the link in the request message is: /api/swap/v3/instruments/BTC-USD-SWAP/ticker
, it is to request the BTC native perpetual contract market data.
POST request message:
POST /api/swap/v3/order HTTP/1.1 Host: www.baidu.com User-Agent: Mozilla/5.0 (Macintosh; Intel Mac OS X 10_9_3) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/35.0.1916.153 Safari/537.36 Content-Length: 25 Content-Type: application/json; charset=UTF-8 Ok-Access-Key: d487230f-ccccc-aaaaa-bbbbb-268fef99cfe4 Ok-Access-Passphrase: abc123 Ok-Access-Sign: h1x6f80rhhkELobJcO1rFyMgUUshOlmgjRBHD+pLvG0= Ok-Access-Timestamp: 2020-09-23T08:43:49.906Z Accept-Encoding: gzip {"aaa":"111","bbb":"222"}
We can see the request path is: /api/swap/v3/order
.
Verified Access Key: d487230f-ccccc-aaaaa-bbbbb-268fef99cfe4
(For demonstration only, not the real KEY)
Signature of the request: h1x6f80rhhkELobJcO1rFyMgUUshOlmgjRBHD+pLvG0=
API KEY secret key--Passphrase: abc123
(For demonstration only)
Requested Body data: {"aaa":"111","bbb":"222"}
。
In this way, we can observe the request message and analyze the reason why the interface request encounters an error.
2. Local listening request
The second method does not need to create a robot, just uses the Netcat
that comes with the Mac: https://baike.baidu.com/item/Netcat/9952751?fr=aladdin. Monitor the requests and print messages.
On the terminal, run Netcat with the command nc - l 8080
.
As the picture below:
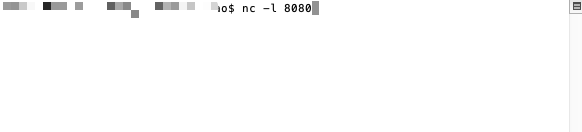
Similarly, we deploy a docker on our computer, and then use the following code to send requests in the debugging tool.
function main(){ exchange.SetBase("http://127.0.0.1:8080") // Here, we change the base address to the local machine, port 8080, and Netcat can get the request // POST request exchange.IO("api", "POST", "/api/swap/v3/order", "aaa=111&bbb=222") // GET request exchange.SetContractType("swap") exchange.GetTicker() }
The POST request message printed out on the terminal:
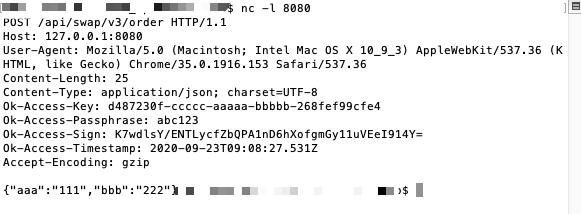
The GET request message printed out on the terminal:
