In the last days, I received some private messages from telegram group users, they hope to have a design example of subscribing new shares strategy for reference. Sometimes, they want to subscribe new shares when the exchange lands new currencis, so in this article, we will design a simple tool strategy for subscribing new shares.
Strategy needs
For example, at present, an exchange and a trading pair: XXX_USDT, has not yet listed on the exchange. But it is going listed soon. We need to follow the XXX_USDT market of this exchange with a program. Once the trading pair is listed, it can be traded. We issue 10 limited price purchase orders, specify the amount, and list the order to subscribe new currencies. If you can buy them successfully, you can complete the task. If not, you can list it until all the orders are closed and you can buy currencies.
The needs are very simple, but for those who have no programming foundation in the digital currency market, they may not be able to start, so let’s get started to implement it.
Strategy code
Strategy parameter definition:
Here we define these 7 parameters to control operations such as placing orders.
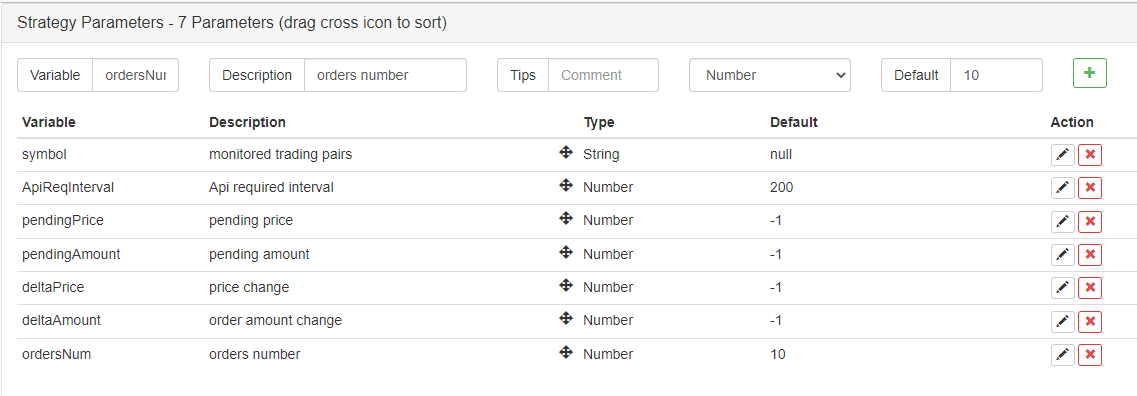
Code implementation:
function pendingOrders(ordersNum, price, amount, deltaPrice, deltaAmount) { var routineOrders = [] var ordersIDs = [] for (var i = 0 ; i < ordersNum ; i++) { var routine = exchange.Go("Buy", price + i * deltaPrice, amount + i * deltaAmount) routineOrders.push(routine) Sleep(ApiReqInterval) } for (var i = 0 ; i < routineOrders.length ; i++) { var orderId = routineOrders[i].wait() if (orderId) { ordersIDs.push(orderId) Log("placed an order successfully", orderId) } } return ordersIDs } function main() { if (symbol == "null" || pendingPrice == -1 || pendingAmount == -1 || pendingPrice == -1 || deltaPrice == -1 || deltaAmount == -1) { throw "Parameter setting error" } exchange.SetCurrency(symbol) // Block error messages SetErrorFilter("GetDepth") while (true) { var msg = "" var depth = exchange.GetDepth() if (!depth || (depth.Bids.length == 0 && depth.Asks.length == 0)) { // No depth msg = "No depth data, wait!" Sleep(500) } else { // Obtain depth Log("Place orders concurrently!") var ordersIDs = pendingOrders(ordersNum, pendingPrice, pendingAmount, deltaPrice, deltaAmount) while (true) { var orders = _C(exchange.GetOrders) if (orders.length == 0) { Log("The current number of pending orders is 0, and the operation is stopped") return } var tbl = { type: "table", title: "The current pending orders", cols: ["id", "price", "amount"], rows: [] } _.each(orders, function(order) { tbl.rows.push([order.Id, order.Price, order.Amount]) }) LogStatus(_D(), "\n`" + JSON.stringify(tbl) + "`") Sleep(500) } } LogStatus(_D(), msg) } }
The strategy checks the exchange API and the order book interface. Once the order book data can be obtained, the strategy will use exchange.Go function to places orders concurrently. After the order is placed, the status of the current pending order will be checked circularly. The strategy has not been actually tested, here is just a code design reference. If you are interested, you can modify or add functions to it.
Complete strategy from: https://www.fmz.com/strategy/358383
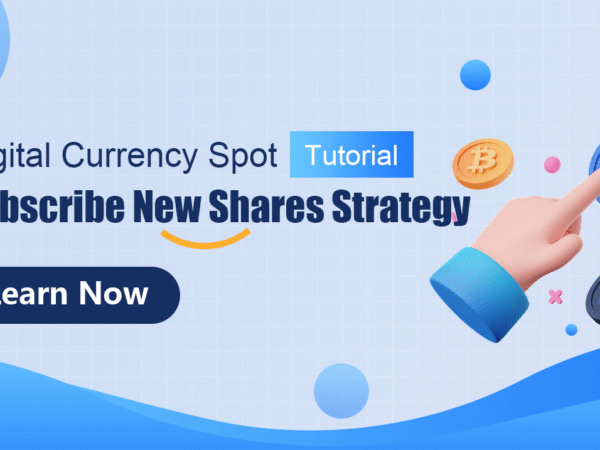